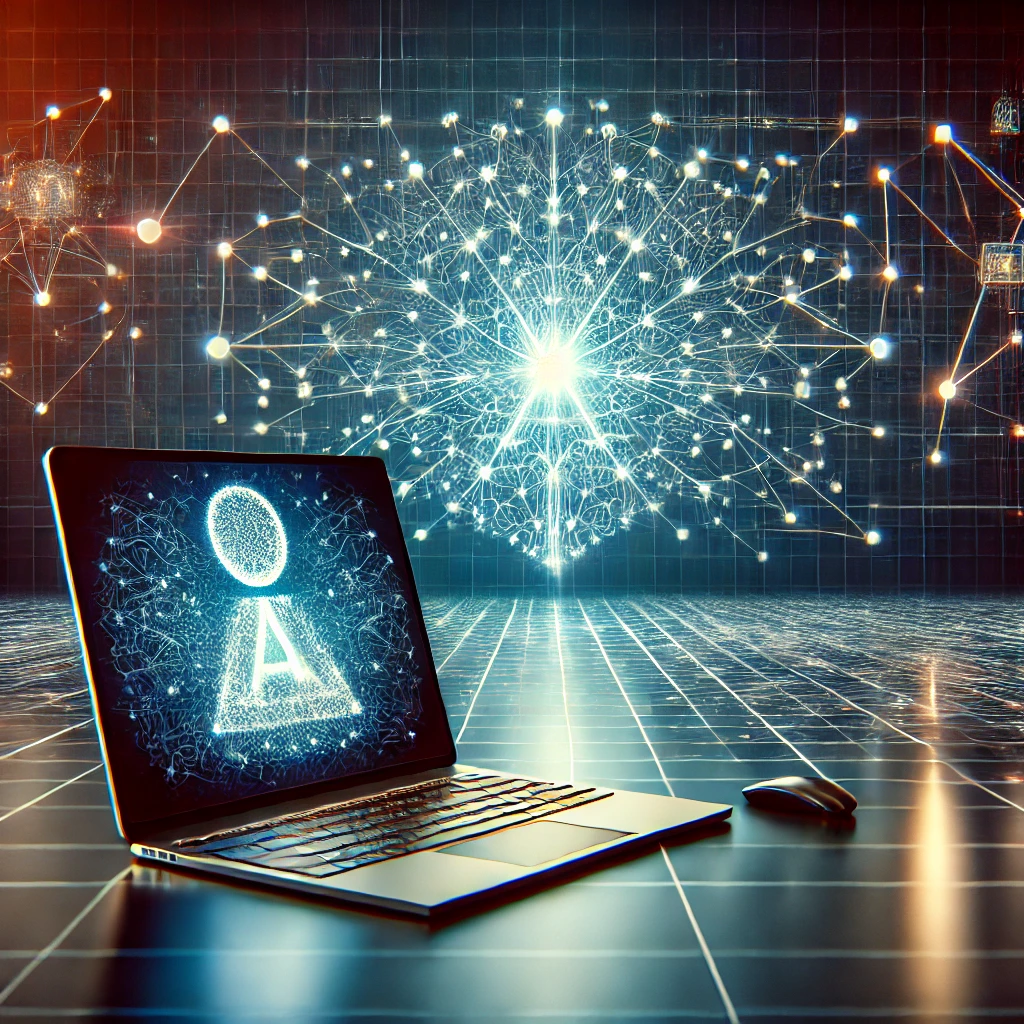
Running a Local AI Model on Ubuntu
Llama 3 Local Setup: Running and Optimizing Performance
This guide covers running Llama 3.1 8B efficiently on your system, including parameter optimization, performance benchmarking, and best practices for different use cases.
Starting the Web Interface
First, let's start the text-generation-webui:
# Navigate to the web UI directory
cd ~/llama3_project/webui/text-generation-webui
# Activate virtual environment
source ~/llama3_project/venv/bin/activate
# Start the server
python server.py
Access the interface at: http://127.0.0.1:7860
Loading the Model
In the web UI:
- Go to the "Model" tab
- Select
Meta-Llama-3.1-8B-Instruct-bnb-4bit
- Click "Load"
Monitor resource usage:
# In a separate terminal
watch -n 1 nvidia-smi
Expected resource usage:
- VRAM: 6-7.5GB
- GPU utilization: ~80% during generation
Optimizing Parameters
Here are recommended parameter presets for different use cases:
Balanced (General Use)
Max new tokens: 300
Temperature: 0.7
Top_p: 0.9
Repetition penalty: 1.1
Batch size: 1
Context length: 2048
Creative Writing
Max new tokens: 500
Temperature: 0.9
Top_p: 0.95
Repetition penalty: 1.05
Context length: 1536
Technical/Accurate
Max new tokens: 200
Temperature: 0.5
Top_p: 0.85
Repetition penalty: 1.2
Context length: 1024
Performance Benchmarking
To benchmark your setup:
- Monitor GPU metrics:
watch -n 1 "nvidia-smi --query-gpu=timestamp,name,temperature.gpu,utilization.gpu,memory.used,memory.total --format=csv"
- Run test prompts with different configurations:
Example benchmark table:
Test Type | Settings | VRAM Usage | Tokens/sec | Notes |
---|---|---|---|---|
Balanced | Default | 6300 MiB | 18 | Good for general use |
Creative | High temp | 7800 MiB | 13 | More diverse outputs |
Technical | Low temp | 6100 MiB | 20 | More focused responses |
Memory Optimization
To optimize memory usage:
Context Length Management:
- Shorter context = less VRAM usage
- Balance between context and performance
Batch Size Adjustment:
- Keep batch size at 1 for 8GB VRAM
- Increase only if VRAM allows
Memory-Saving Techniques:
# Add to your startup script
import torch
torch.cuda.empty_cache()
Use Case Optimization
For Long Conversations
Context length: 2048
Max new tokens: 300
Temperature: 0.7
Top_p: 0.9
For Code Generation
Context length: 1024
Max new tokens: 200
Temperature: 0.4
Top_p: 0.95
For Creative Writing
Context length: 1536
Max new tokens: 500
Temperature: 0.9
Top_p: 0.95
Performance Monitoring
Real-time Monitoring
- GPU Usage:
nvidia-smi dmon -i 0
- System Resources:
htop
Performance Metrics to Watch
- VRAM usage (should stay under 7.5GB)
- GPU utilization (optimal: 70-90%)
- Generation speed (tokens/second)
- Temperature impact on response quality
Troubleshooting Performance Issues
High VRAM Usage
Solutions:
- Reduce context length
- Clear VRAM between runs
- Close background applications
Slow Generation
Solutions:
- Reduce max new tokens
- Optimize temperature settings
- Check for system bottlenecks
Quality Issues
Solutions:
- Adjust temperature and top_p
- Fine-tune repetition penalty
- Experiment with different presets
Best Practices
Regular Maintenance:
- Clear VRAM between long sessions
- Monitor system temperatures
- Update drivers regularly
Parameter Management:
- Save successful configurations
- Document performance metrics
- Create task-specific presets
Resource Management:
- Close unnecessary applications
- Monitor system resources
- Plan for cooling during long sessions
Advanced Optimization
Custom Scripts
Create a startup script with optimized settings:
# optimize_llama.py
import torch
import gc
def optimize_memory():
torch.cuda.empty_cache()
gc.collect()
return torch.cuda.memory_allocated()
def set_optimal_parameters(model, task_type="balanced"):
params = {
"balanced": {
"max_tokens": 300,
"temperature": 0.7,
"top_p": 0.9
},
"creative": {
"max_tokens": 500,
"temperature": 0.9,
"top_p": 0.95
},
"technical": {
"max_tokens": 200,
"temperature": 0.5,
"top_p": 0.85
}
}
return params[task_type]
## Creating Custom Configuration Profiles
You can save different configuration profiles for various use cases:
1. Create a profiles directory:
```bash
mkdir ~/llama3_project/webui/text-generation-webui/profiles
- Create JSON configuration files:
{
"creative_writing": {
"max_new_tokens": 500,
"temperature": 0.9,
"top_p": 0.95,
"repetition_penalty": 1.05,
"context_length": 1536
}
}
- Load profiles through the web UI or command line arguments.
Scheduling and Automation
For regular usage patterns, consider automating model management:
- Create a startup script:
#!/bin/bash
# start_llama.sh
# Activate virtual environment
source ~/llama3_project/venv/bin/activate
# Clear GPU memory
python -c "import torch; torch.cuda.empty_cache()"
# Start the web UI with specific parameters
python server.py --model Meta-Llama-3.1-8B-Instruct-bnb-4bit --gpu-memory 7000
- Make it executable:
chmod +x start_llama.sh
Long-term Performance Maintenance
Daily Maintenance
- Clear VRAM before starting new sessions
- Monitor GPU temperatures
- Check system resources
Weekly Maintenance
- Update the web UI
- Review and optimize configurations
- Back up custom profiles
Monthly Maintenance
- Check for model updates
- Review and update parameter settings
- Analyze performance metrics
- Clean up old log files
Conclusion
Running Llama 3.1 8B efficiently on an RTX 4070 requires careful balance of parameters and regular maintenance. By following these optimization guidelines and maintaining good practices, you can achieve reliable performance for various use cases.
Remember to:
- Monitor resource usage regularly
- Adjust parameters based on your specific needs
- Maintain system health
- Document successful configurations
- Update and optimize regularly
With these optimizations in place, you should have a stable and efficient local AI model ready for your projects.
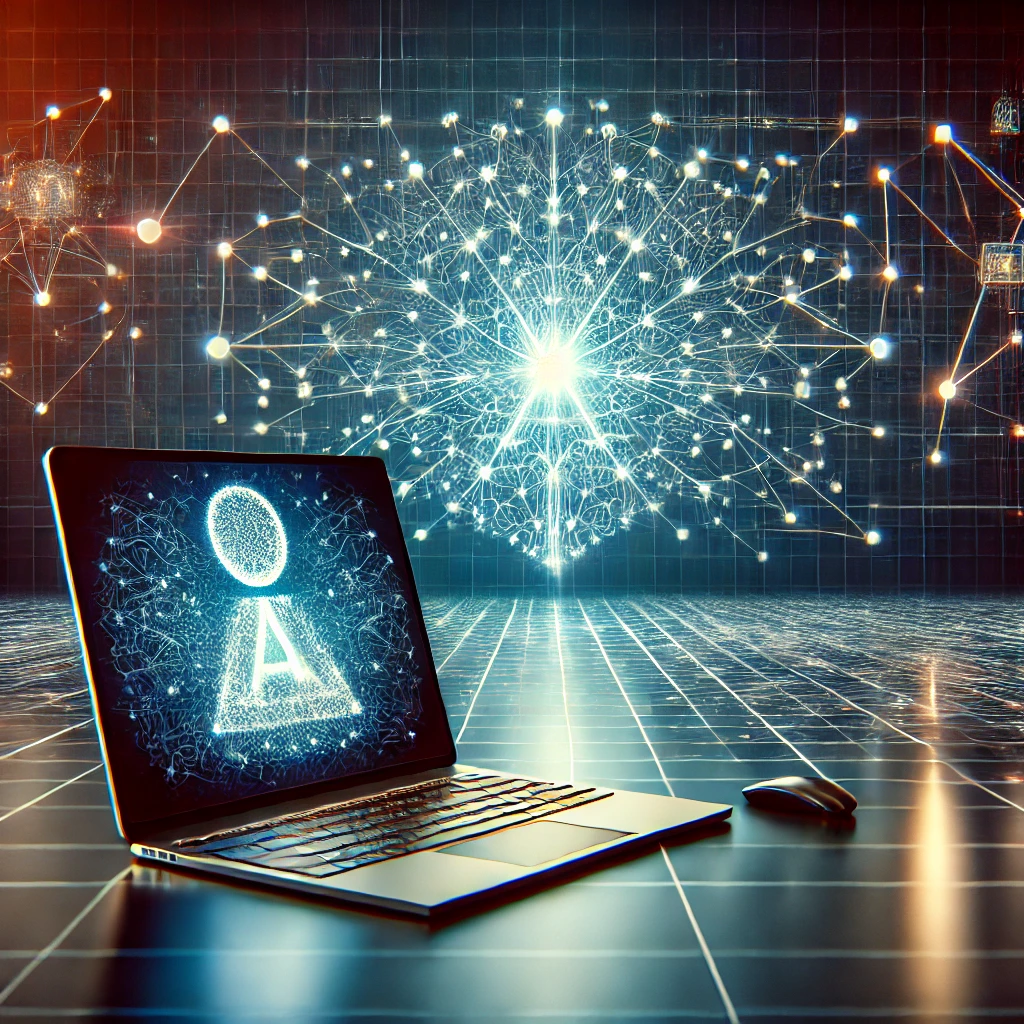