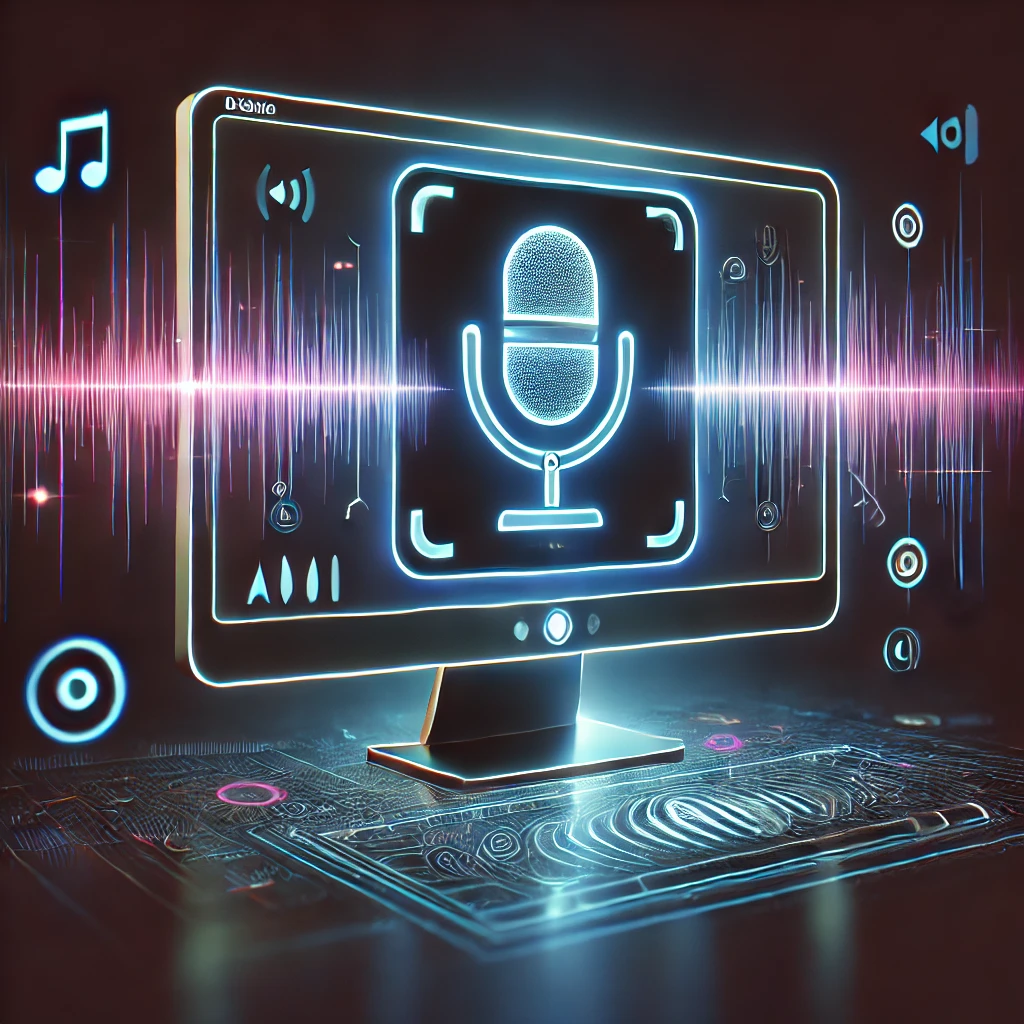
AI-Powered Speech-to-Text Web App
AI Speech-to-Text Web App: Setting Up the Environment
In this guide, we'll set up the development environment for our AI-powered speech-to-text web application. We'll create a virtual environment, install all necessary dependencies, and prepare our project structure.
Creating a Project Directory
First, let's create a dedicated directory for our project:
# Create a new project directory
mkdir speech-to-text-app
cd speech-to-text-app
Setting Up a Python Virtual Environment
Virtual environments allow us to create isolated spaces for Python projects, ensuring dependencies for different projects don't interfere with each other. Let's create one for our project:
# Create a virtual environment
python -m venv venv
# Activate the virtual environment
# On Windows:
venv\Scripts\activate
# On macOS/Linux:
source venv/bin/activate
You should see the name of your virtual environment in parentheses at the beginning of your command prompt, indicating that it's active.
Installing Required Packages
Now we'll install all the packages needed for our application:
# Upgrade pip
pip install --upgrade pip
# Install core packages
pip install flask openai-whisper pyaudio numpy
# Install additional packages for web interface
pip install flask-bootstrap
Let's explain what each package does:
- Flask: A lightweight web framework for Python
- openai-whisper: OpenAI's speech recognition model
- PyAudio: For capturing audio from your microphone
- NumPy: A library for numerical computations in Python
- Flask-Bootstrap: Integration of Bootstrap with Flask
Troubleshooting PyAudio Installation
PyAudio can sometimes be challenging to install. If you encounter issues, try these platform-specific solutions:
On Windows:
pip install pipwin
pipwin install pyaudio
On macOS:
brew install portaudio
pip install pyaudio
On Linux (Ubuntu/Debian):
sudo apt-get install python3-pyaudio
Creating a Requirements File
Let's create a requirements.txt file to make it easier to install all dependencies in the future:
pip freeze > requirements.txt
This command creates a file listing all the packages and their versions installed in your virtual environment.
Setting Up the Project Structure
Now, let's create the basic structure for our Flask application:
# Create project directories
mkdir -p static/js static/css templates
# Create main application file
touch app.py
# Create additional files
touch templates/index.html
touch static/js/main.js
touch static/css/style.css
Our project structure should now look like this:
speech-to-text-app/
├── venv/ # Virtual environment
├── static/ # Static files
│ ├── css/ # CSS files
│ │ └── style.css # Custom styles
│ └── js/ # JavaScript files
│ └── main.js # Custom JavaScript
├── templates/ # HTML templates
│ └── index.html # Main page template
├── app.py # Main Flask application
└── requirements.txt # Package dependencies
Testing the Environment
Let's create a simple Flask application to test our setup:
# app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
And a basic HTML template:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>AI Speech-to-Text App</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}" />
</head>
<body>
<h1>Speech-to-Text Application</h1>
<p>Environment setup successful!</p>
<script src="{{ url_for('static', filename='js/main.js') }}"></script>
</body>
</html>
Now, let's run the application to test our setup:
python app.py
Open your web browser and navigate to http://127.0.0.1:5000/
. You should see a page with the heading "Speech-to-Text Application" and a message indicating that the environment setup was successful.
Installing the Whisper Model
Finally, let's download a smaller version of the Whisper model to ensure it runs efficiently on standard hardware:
# In a Python interpreter or script
import whisper
model = whisper.load_model("base")
This will download the base model, which is about 142MB. The base model offers a good balance between accuracy and resource requirements.
Conclusion
In this guide, we've:
- Created a virtual environment for our project
- Installed all necessary dependencies
- Set up the basic structure for our Flask application
- Verified that our environment is working correctly
- Downloaded the Whisper model
Now that our environment is set up, we're ready to start building the speech-to-text application itself. In the next guide, we'll create the necessary files and write the code to make our application work.
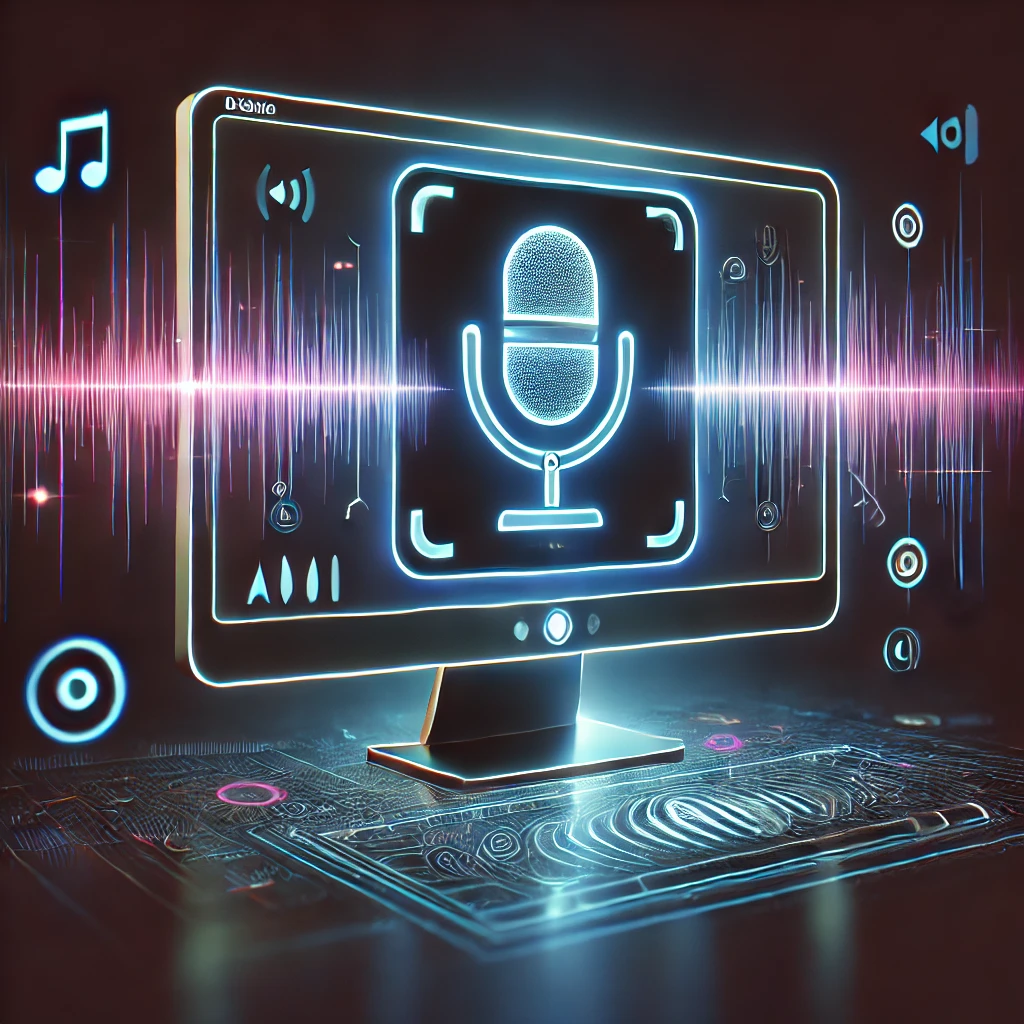